- What are you? null, undefined – or just nullish? - 22. Oktober 2021
- AI-based Code Autocompletion with TabNine - 13. Juli 2021
- Building a Home Office Challenges App with Kafka, Kubernetes and StencilJS – Part 2: Introduction to Apache Kafka - 18. Januar 2021
Whether you’re new to programming or already an expert, I’m sure you’re already familiar with null references, a common feature of most programming languages. If you’re not, feel free to take a short look at this definition before we go on:
In computing, a null pointer or null reference is a value saved for indicating that the pointer or reference does not refer to a valid object.
Wikipedia
See, that wasn’t so difficult, was it? It probably won’t surprise you to hear that you can find these null references in JavaScript, too. Well, kind of, because in JS, they are quite different, and rather confusing. So, let’s clear things up a bit.
What does it all mean?
JS doesn’t just have null, but it also has undefined. Both null and undefined indicate that there is no value. But here’s where things get complicated.
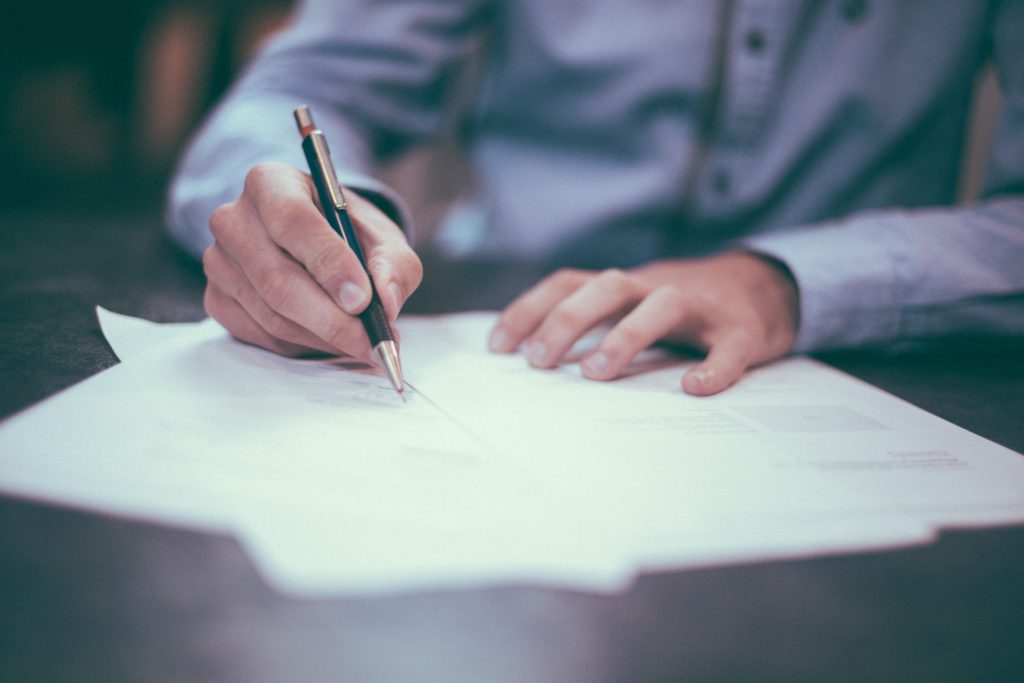
Imagine you’re doing some paperwork and filling out a form. But there’s one field that you can’t fill out because you don’t know what to put there. Or perhaps you don’t want to provide that information. Do you leave the field blank? Or do you write something like ‘not applicable’? And why is that important? Aren’t they both the same?
Now image that you’re the person reading the form, and you encounter a field which says ‘not applicable’. In that case, you can assume that the person saw the field, thought about it, and then chose not to provide an answer.
But what if the field was left blank? Did the person not see the field? Did they forget to fill it out? Or did they leave it blank on purpose? Nobody knows.
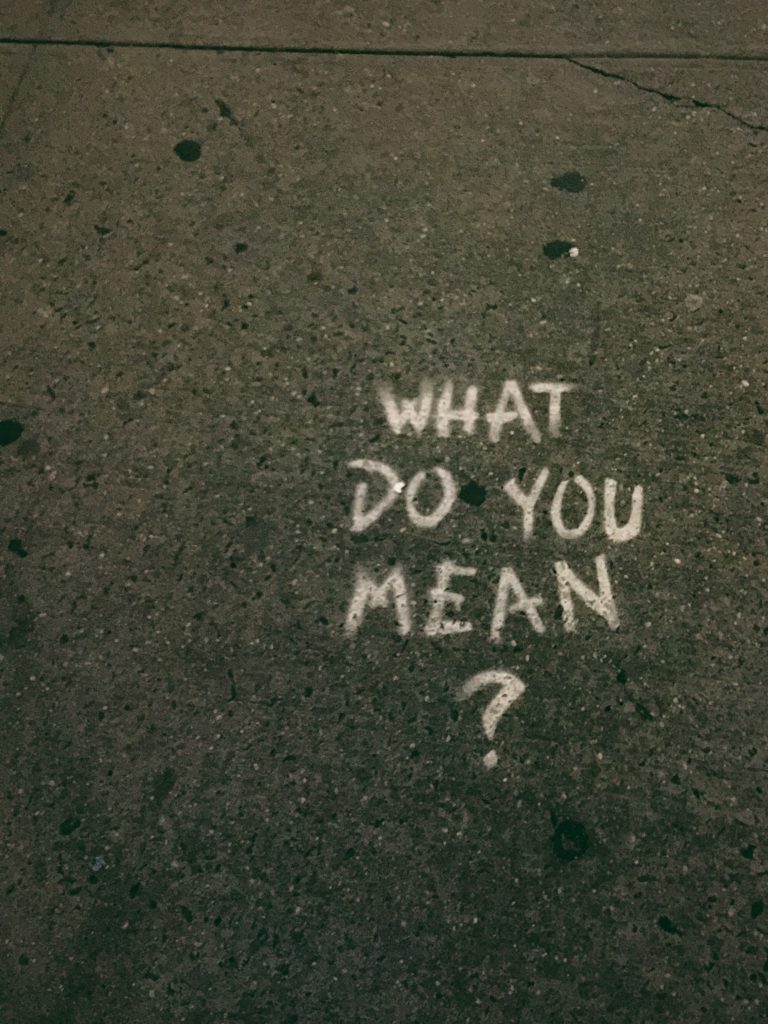
In JavaScript, the equivalent to a blank field is undefined. A value is absent, and we don’t know why. The equivalent to ‘not applicable’ is null.
Perhaps it is helpful if we take a look at the official definitions in the ECMAScript2015 specification:
undefined: primitive value used when a variable has not been assigned a value
null: primitive value that represents the intentional absence of any object value
The important term here is the ‘intentional’. If you set a value to null, you’re telling the program (and everyone reading the code) that this variable should not have a value (yet). Anyone reading your code will be able to understand.
On the other hand, if a value is still undefined, it means that nobody has bothered to assign a value to it. Why that is the case, is anyone’s guess.
When do you encounter undefined?
There’s a rather long list of reasons why you could have to deal with undefined in your code. The most obvious case is, of course, when a variable has been already declared, but not been assigned a value. But here are a few of the other most common situations:
- You’re trying to access a certain property on an object, but that property is missing.
- You’re trying to access a specific element in an array by its index, but there is no such element at the given position.
- You’re trying to access the return value of a function, but the function is missing a return statement.
- You’re trying to access a parameter from within a function, but no corresponding argument is passed to the function.
Different but equal
In the context of JavaScript, the following expression is true:
null == undefined
However, if we use the strict equality operator (===) instead, which also includes a type comparison, the result will be false.
That is because null und undefined have different types. If a variable is undefined, it will also have the type undefined. If a variable has the value null, its type will be object. (It isn’t really an object, but that’s a story for another time, or another blog.)
So, if you want to handle some value which could either be null or undefined, you should better check for both. If you have to use the strict equality operator, this mean that you have to do two checks every single time, which can be quite annoying.
Of course, there’s also a way to check for null and undefined both at the same time:
if (value == null) {
// Do something!
}
We can also check if a value is null or undefined with the nullish coalescing operator (??). In case you’re wondering what that strange term ‘nullish’ is supposed to mean, the answer is quite simple: If something is nullish, it’s either null or undefined.
Conclusion
As we have learned, using null is a good practice if you want to make clear that you intentionally chose not to set a specific value.
Having said that, there are people who believe that null references should not be used at all in the context of Object-Oriented Programming. Even their own inventor considers them to be a Billion Dollar Mistake.
Still others believe that one should only use undefined in JavaScript, and want to disallow the usage of null altogether.
At the end, it’s up to you to figure out for yourself which approach works best for your code base and the people and APIs you work with.